Building Scalable Laravel Apps
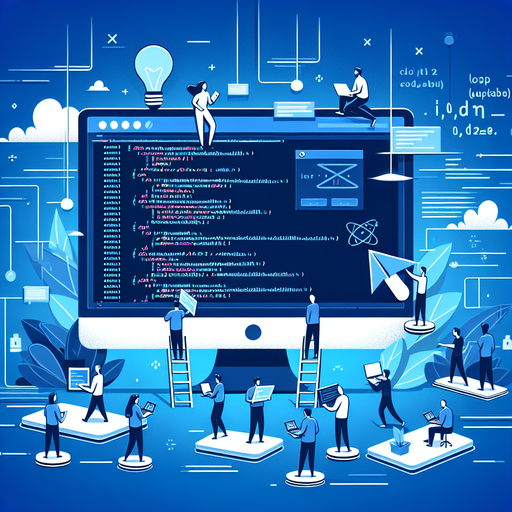
Contenidos
Introduction to Scalable Laravel Apps
In the world of web development, scalability plays a crucial role in ensuring the success of an application. When it comes to Laravel development, building scalable apps is of utmost importance to accommodate growing user bases, increasing data volumes, and evolving business needs. This section provides an introduction to scalable Laravel apps, highlighting the importance and benefits associated with this approach.
Importance of Scalability in Laravel Development
Scalability is the ability of an application to handle increased workload and growing demands without compromising performance or user experience. In Laravel development, scalability is vital to meet the needs of businesses that anticipate growth or experience sudden spikes in traffic. By building scalable Laravel apps, developers can ensure that the application remains responsive, reliable, and efficient as the user base expands.
Scalable Laravel apps offer several advantages, such as improved user satisfaction, reduced downtime, and increased revenue potential. When an application can handle a higher volume of concurrent users and process data efficiently, users experience faster response times and seamless interactions. This positive user experience fosters customer loyalty and encourages repeat visits. Additionally, with reduced downtime resulting from system overload, businesses can avoid revenue loss and maintain customer trust.
Benefits of Building Scalable Laravel Apps
Building scalable Laravel apps comes with a range of benefits that contribute to the long-term success and sustainability of the application. Some of the key advantages include:
- Flexibility: Scalable Laravel apps are designed to adapt to changing requirements and accommodate future growth. The modular nature of Laravel enables developers to add new features, make enhancements, and scale the application as needed without rewriting the entire codebase.
- Improved Performance: Scalable Laravel apps are optimized for performance, ensuring that the application remains responsive even under heavy load. By implementing caching mechanisms, optimizing database queries, and utilizing queues for asynchronous processing, developers can enhance performance and deliver a seamless user experience.
- Cost Efficiency: Scalability allows businesses to optimize their infrastructure and resources, ensuring that the application runs efficiently without unnecessary overhead. By implementing horizontal scaling or load balancing techniques, businesses can distribute the workload across multiple servers, reducing the need for expensive hardware upgrades.
- Future-Proofing: Building scalable Laravel apps future-proofs the application by ensuring that it can handle growth and evolving business needs. As technology advances and user demands change, a scalable Laravel app can easily adapt and incorporate new features, integrations, and functionalities without compromising stability.
By recognizing the importance of scalability in Laravel development and harnessing its benefits, businesses can revolutionize their development practices and deliver applications that can grow and evolve alongside their user base. The subsequent sections will dive deeper into the planning, implementation, and continuous improvement aspects of building scalable Laravel apps.
Planning for Scalability
To build scalable Laravel applications, proper planning is essential. This section focuses on understanding your application’s requirements and designing a scalable architecture that can accommodate future growth.
Understanding Your Application’s Requirements
Before diving into the development process, it’s crucial to have a clear understanding of your application’s requirements. This involves analyzing factors such as anticipated user base, expected traffic volume, and potential future functionalities. By gathering this information, you can make informed decisions about the infrastructure and architecture needed to support scalability.
Consider the following aspects when understanding your application’s requirements:
- User Base: Determine the number of concurrent users your application is expected to handle. This will influence the resources required to ensure optimal performance.
- Traffic Volume: Evaluate the expected traffic volume, including peak periods, to estimate the server capacity needed. Consider factors like user interactions, data processing, and file storage requirements.
- Functionality: Identify the core features your application must support and any potential future enhancements. Understanding the application’s functionality will help determine the scalability requirements for both the front-end and back-end components.
By thoroughly understanding your application’s requirements, you can make informed decisions about the infrastructure, technologies, and strategies required to build a scalable Laravel application.
Designing a Scalable Architecture
Once you have a clear understanding of your application’s requirements, it’s time to design a scalable architecture. A well-designed architecture ensures that your Laravel application can handle increased workload and user demands without sacrificing performance.
Consider the following practices when designing a scalable architecture:
- Separation of Concerns: Implement a modular approach where different components of your application have specific responsibilities. This allows for easier maintenance, scalability, and code reusability.
- Scalable Database Design: Design your database schema to handle increasing amounts of data efficiently. Utilize techniques like database indexing, normalization, and denormalization, depending on your specific use cases.
- Caching: Implement caching mechanisms to reduce the load on your database and improve response times. Utilize tools like Redis or Memcached to cache frequently accessed data or query results.
- Horizontal Scaling: Consider a horizontally scalable architecture, where you can add more servers to distribute the workload. This approach allows for improved performance and higher availability. For more information on horizontal scaling, check out our article on overview-laravel-custom-development.
- Load Balancing: Implement load balancing techniques to evenly distribute incoming traffic across multiple servers. This ensures that no single server becomes overwhelmed and helps maintain consistent performance even during peak traffic periods. Learn more about load balancing in our article on laravel-project-management.
By designing a scalable architecture that aligns with your application’s requirements, you can lay a solid foundation for the development of a Laravel application capable of handling increased demands as your business grows.
Implementing Best Practices
To build scalable Laravel apps, it’s crucial to implement best practices that optimize performance and efficiency. In this section, we will explore three key practices: utilizing caching mechanisms, optimizing database queries, and implementing queues for asynchronous processing.
Utilizing Caching Mechanisms
Caching is an effective technique to improve the performance of your Laravel app. By storing frequently accessed data in cache, you can reduce the need for repetitive and resource-intensive operations, resulting in faster response times.
Laravel provides built-in support for various caching mechanisms, including file-based caching, database caching, and in-memory caching using popular solutions like Redis and Memcached. These caching mechanisms help to alleviate the load on the database and enhance the overall speed and scalability of your application.
By implementing appropriate caching strategies for different parts of your application, such as query results, computed data, or rendered views, you can significantly reduce the time required to serve subsequent requests. For more information on how to leverage caching in Laravel, refer to our article on laravel custom development.
Optimizing Database Queries
Database queries can be a major bottleneck in application performance, especially in scenarios where complex relationships and large datasets are involved. Optimizing your database queries is essential for improving the scalability of your Laravel app.
To optimize database queries, consider the following techniques:
- Use eager loading: Utilize Laravel’s eager loading feature to load related models in a single database query, reducing the number of round-trips to the database.
- Indexing: Ensure that your database tables have appropriate indexes on columns frequently used in search, join, and sorting operations. This helps to speed up query execution.
- Select only required columns: Fetch only the columns that are necessary for a particular operation, instead of retrieving the entire row. This minimizes unnecessary data transfer and improves performance.
- Consider pagination: When dealing with large result sets, implement pagination to retrieve data in smaller chunks. This prevents the application from unnecessarily loading and rendering a large amount of data at once.
By optimizing your database queries, you can minimize the load on the database server and improve the overall responsiveness of your Laravel app.
Implementing Queues for Asynchronous Processing
Queues allow you to perform time-consuming and resource-intensive tasks asynchronously, improving the responsiveness and scalability of your Laravel app. By offloading tasks to a queue, your application can quickly respond to user requests without being blocked by time-consuming operations.
Laravel’s queue system provides support for various queue drivers, including Redis, Beanstalkd, and Amazon SQS. By configuring a queue driver and defining jobs, you can easily handle tasks such as sending emails, processing large files, or performing complex calculations in the background.
By leveraging queues, you can ensure that your Laravel app remains performant and responsive, even under heavy loads. For more information on implementing queues in Laravel, refer to our article on laravel project management.
By utilizing caching mechanisms, optimizing database queries, and implementing queues for asynchronous processing, you can enhance the performance and scalability of your Laravel app. These best practices work together to reduce the load on your application, improve response times, and provide a seamless user experience.
Scaling Your Laravel App
When it comes to building scalable Laravel apps, scaling is a crucial aspect to consider. Scaling refers to the ability of an application to handle increased workload and accommodate growth. In this section, we will explore two important concepts in scaling Laravel apps: horizontal scaling vs. vertical scaling and load balancing for high traffic scenarios.
Horizontal Scaling vs. Vertical Scaling
When scaling a Laravel app, you have two primary options: horizontal scaling and vertical scaling. Let’s take a closer look at each approach:
Horizontal Scaling: Horizontal scaling involves adding more servers or instances to your application’s infrastructure to handle increased traffic and workload. With horizontal scaling, you distribute the load across multiple servers, allowing for improved performance and capacity. This approach is particularly useful when your application experiences a surge in traffic or requires high availability.
Vertical Scaling: Vertical scaling, on the other hand, involves upgrading the resources of your existing server, such as CPU, RAM, or disk space, to handle increased workload. This approach allows your application to handle more requests by improving the capabilities of a single server. Vertical scaling is suitable when your application needs to handle a moderate increase in traffic or workload.
Deciding between horizontal and vertical scaling depends on various factors, including your application’s specific requirements, budget, and future growth plans. It’s important to assess the scalability needs of your Laravel app and choose the most appropriate scaling approach accordingly.
Load Balancing for High Traffic
In scenarios where your Laravel app experiences high traffic, load balancing can be an effective strategy to ensure optimal performance and availability. Load balancing involves distributing incoming network traffic across multiple servers to prevent any single server from becoming overwhelmed.
Load balancers act as intermediaries between users and your application servers, intelligently routing requests to the server with the least load or based on predefined algorithms. This helps to evenly distribute the workload and improve response times.
Implementing load balancing for your Laravel app can provide several benefits, including improved scalability, increased reliability, and enhanced fault tolerance. By distributing the traffic across multiple servers, load balancing enables your application to handle a higher number of concurrent requests and ensures that your users experience a smooth and responsive application.
To implement load balancing, you can utilize various load balancing techniques, such as round-robin, least connections, or weighted distribution. The choice of load balancing technique depends on your specific requirements and infrastructure setup.
By considering horizontal scaling, vertical scaling, and load balancing techniques, you can ensure that your Laravel app is capable of handling increased traffic and growing user demands. Remember to regularly monitor your application’s performance and make necessary adjustments to maintain optimal scalability. For more information on Laravel custom development, check out our article on overview-laravel-custom-development.
Monitoring and Testing
To ensure the scalability of your Laravel application, it’s crucial to implement monitoring tools and conduct load testing for scalability verification. These practices play a vital role in identifying bottlenecks, optimizing performance, and ensuring a smooth user experience.
Implementing Monitoring Tools
Monitoring tools allow you to keep a close eye on the performance and health of your Laravel application. By tracking key metrics and monitoring system resources, you can proactively detect any issues that may impact scalability. Some common monitoring tools used in Laravel development include:
- Application Performance Monitoring (APM) Tools: APM tools provide detailed insights into the performance of your application. They help identify slow database queries, inefficient code, and other performance bottlenecks. Examples of popular APM tools for Laravel include New Relic, Datadog, and Blackfire.
- Logging and Error Tracking: Implementing a robust logging and error tracking system is essential for monitoring your application’s health. Tools like Laravel LogViewer and Sentry can help you track errors, exceptions, and log messages, allowing you to quickly identify and resolve issues.
- Server Monitoring: Monitoring the server resources is crucial to ensure optimal performance. Tools like Nagios, Zabbix, or Datadog can help you monitor CPU usage, memory usage, disk space, and other server metrics.
By implementing these monitoring tools, you can gain valuable insights into your application’s performance, detect potential issues, and take proactive measures to optimize scalability. For more information on Laravel custom development, refer to our article on overview-laravel-custom-development.
Conducting Load Testing for Scalability Verification
Load testing is a critical step in verifying the scalability of your Laravel application. It involves simulating real-world scenarios and subjecting your application to varying levels of user traffic and system load. Load testing helps identify the performance limits of your application and ensures that it can handle increased traffic and user demand.
Here are some key steps to conduct load testing for scalability verification:
- Identify Performance Metrics: Determine the performance metrics you want to monitor during load testing. This may include response time, throughput, error rates, and resource utilization.
- Create Realistic Test Scenarios: Define realistic user scenarios that replicate the expected load on your application. Consider factors such as concurrent users, transaction volumes, and user behavior patterns.
- Select Load Testing Tools: Choose a suitable load testing tool such as Apache JMeter, Gatling, or Locust. These tools allow you to simulate user traffic, generate load, and measure application performance under different scenarios.
- Execute Load Tests: Run the load tests using the defined scenarios and monitor the performance metrics. Analyze the results to identify any performance bottlenecks, such as slow response times or high error rates.
- Optimize and Retest: Based on the load test results, optimize your application by addressing any performance issues. Make necessary code optimizations, employ caching mechanisms, or scale up the infrastructure as required. Retest the application to ensure the optimizations have improved its scalability.
Load testing provides valuable insights into your application’s scalability and helps you fine-tune its performance. By conducting regular load tests, you can identify potential issues early on and ensure that your Laravel application can handle increased user demand. For more information on Laravel development and project management, refer to our articles on laravel-project-management and barcelona-laravel-custom-development.
Continuous Improvement
To ensure the long-term success and scalability of your Laravel application, it’s important to prioritize continuous improvement. This involves ongoing efforts to refine and optimize your codebase, as well as staying up-to-date with the latest updates and advancements in Laravel.
Refactoring for Scalability
As your Laravel application evolves and grows, it’s essential to periodically assess and refactor your codebase for scalability. Refactoring involves restructuring your code to improve its maintainability, performance, and scalability without altering its external behavior.
When refactoring for scalability, consider the following:
- Modularization: Break your code into smaller, more manageable modules. This allows for easier maintenance and scalability as you can work on different components independently.
- Optimization: Identify and optimize any bottlenecks in your code. This may involve improving database queries, optimizing algorithms, or implementing caching mechanisms to reduce the load on your servers.
- Scalable Architecture: Ensure that your code follows best practices for building scalable Laravel applications. Design your architecture in a way that allows for horizontal scaling, where you can add more servers to handle increased traffic, as well as vertical scaling, where you can upgrade the resources of existing servers. For more information on designing a scalable architecture, refer to our article on laravel-project-management.
By regularly refactoring your codebase, you can enhance its scalability, maintainability, and performance, allowing your Laravel application to handle increasing demands.
Keeping Up with Laravel Updates
Laravel is a dynamic framework that constantly evolves to meet the changing needs of developers. It’s crucial to stay updated with the latest releases and updates in order to take advantage of new features, improvements, and security patches.
When keeping up with Laravel updates, consider the following:
- Release Notes: Read the release notes for each new version of Laravel to understand the changes and improvements made. This will help you identify any potential compatibility issues with your existing codebase and plan for necessary updates.
- Dependency Updates: Keep track of the dependencies used in your Laravel application, such as packages and libraries. Regularly update these dependencies to their latest versions, ensuring compatibility with the latest Laravel release and taking advantage of any bug fixes or performance enhancements.
- Testing and QA: Before implementing Laravel updates in your production environment, thoroughly test them in a staging or development environment. This helps to identify and resolve any issues or conflicts that may arise due to the updates.
By staying up-to-date with Laravel updates, you can take advantage of new features, improve the security and performance of your application, and ensure compatibility with the evolving Laravel ecosystem.
Continuous improvement is a vital aspect of building scalable Laravel applications. By regularly refactoring your codebase and keeping up with Laravel updates, you can ensure that your application remains efficient, secure, and adaptable to meet the growing needs of your users.