Nuxt.js Custom API Development Made Easy
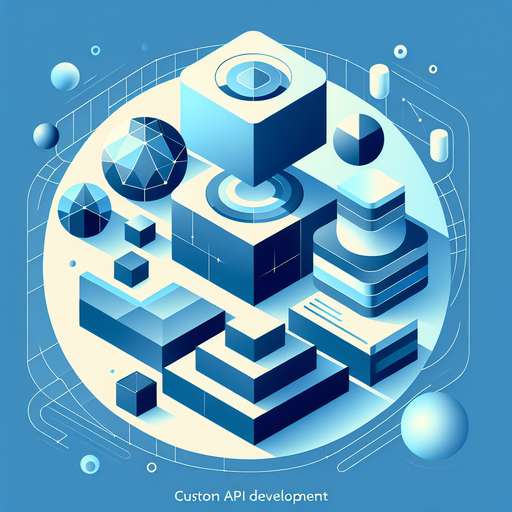
Contenidos
Introduction to Nuxt.js Custom API Development
When it comes to building robust web applications, Nuxt.js is a powerful framework that offers a range of features and benefits. One of the key strengths of Nuxt.js is its ability to seamlessly integrate with custom APIs, allowing developers to create dynamic and interactive web experiences. In this section, we will explore the basics of Nuxt.js and the importance of custom API development in the context of Nuxt.js.
Understanding the Basics of Nuxt.js
Nuxt.js is a framework built on top of Vue.js, a popular JavaScript framework for building user interfaces. It extends Vue.js by providing additional features and conventions that make it easier to develop scalable and performant web applications. Nuxt.js follows the principles of server-side rendering (SSR), which means that the initial rendering of the application is done on the server, improving the overall performance and SEO-friendliness of the application.
By leveraging Nuxt.js, developers can benefit from its modular architecture, automatic routing, and pre-rendering capabilities. These features enable rapid development and enhance the user experience by reducing the time required to load pages.
Importance of Custom API Development
Custom API development plays a vital role in Nuxt.js applications as it enables seamless integration with backend systems and external services. By developing custom APIs, developers have the flexibility to design and implement endpoints that cater specifically to the needs of their application. This allows for efficient data retrieval and manipulation, making the application more dynamic and interactive.
The advantages of custom API development in Nuxt.js are numerous. It allows for decoupling the frontend and backend, enabling independent development and scalability. Custom APIs also provide the ability to aggregate data from multiple sources, making it easier to manage complex data structures and reduce the load on the frontend. Additionally, custom APIs facilitate easier integration with third-party services, such as payment gateways or social media platforms.
To develop custom APIs in Nuxt.js, developers can utilize various technologies and frameworks, such as Express.js or Fastify. These frameworks provide a solid foundation for building RESTful APIs or GraphQL endpoints. By following best practices and employing proper authentication and authorization mechanisms, developers can ensure the security and integrity of their custom APIs.
In the next section, we will explore the process of setting up a Nuxt.js project and discuss the project structure in more detail. Stay tuned to learn how to kickstart your Nuxt.js journey!
Setting Up Your Nuxt.js Project
Before diving into custom API development with Nuxt.js, it’s essential to set up your project properly. This section will guide you through the process of installing Nuxt.js and understanding the project structure.
Installing Nuxt.js
To begin, you need to install Nuxt.js on your development environment. Nuxt.js is built on top of Vue.js and provides a framework for server-side rendering, static site generation, and more. Follow these steps to install Nuxt.js:
- Ensure you have Node.js installed on your system. Nuxt.js requires Node.js version 10.13 or later. You can download Node.js from the official website.
- Open your command-line interface and navigate to the directory where you want to create your Nuxt.js project.
- Run the following command to create a new Nuxt.js project:
npx create-nuxt-app <project-name>
Replace <project-name>
with the desired name for your project.
- You will be prompted to choose a package manager (npm or yarn) and select the features you want to include in your project. Make your selections based on your project requirements.
- Once the installation is complete, navigate into your project directory:
cd <project-name>
Congratulations! You have successfully installed Nuxt.js and set up your project.
Project Structure in Nuxt.js
Understanding the project structure in Nuxt.js will help you navigate your project and locate the necessary files for custom API development. Here is an overview of the key directories and files in a typical Nuxt.js project:
assets/
: This directory contains your uncompiled assets such as CSS, images, and fonts.components/
: Here, you can store your Vue.js components that are specific to your project.layouts/
: This directory contains the layout files for your application. Layouts define the overall structure and design of your pages.pages/
: The pages directory is where you create your application’s views. Each.vue
file in this directory represents a page that can be accessed via its URL.plugins/
: You can place your custom plugins in this directory. Plugins are used to add functionality or configure third-party libraries.static/
: The static directory is where you can place static files such as images or JSON files. These files will be served directly without any special processing.store/
: The store directory is used for Vuex, Nuxt.js’ state management solution. You can organize your store modules in this directory for managing your application’s state.nuxt.config.js
: This file contains the configuration for your Nuxt.js project. You can modify various settings such as modules, plugins, and build options.
By familiarizing yourself with the project structure, you can easily locate and work with the necessary files for custom API development in Nuxt.js.
Now that you have Nuxt.js installed and understand the project structure, you’re ready to dive into creating custom APIs for your Nuxt.js project. Let’s explore the benefits and steps involved in custom API development in the next section.
Creating Custom APIs
In Nuxt.js, creating custom APIs is a powerful way to extend the functionality of your application and interact with external data sources. Custom APIs provide flexibility and control over the data flow in your Nuxt.js project. Let’s explore the benefits of custom APIs in Nuxt.js and the steps to develop them.
Benefits of Custom APIs in Nuxt.js
Custom APIs offer several advantages when developing applications with Nuxt.js:
- Data Integration: Custom APIs allow you to seamlessly integrate data from various sources into your Nuxt.js project. This could include external APIs, databases, or any other data repositories.
- Flexibility: With custom APIs, you have complete control over how the data is fetched, processed, and manipulated. You can customize the API endpoints, implement complex logic, and transform data according to your specific requirements.
- Efficiency: By creating custom APIs, you can optimize the data retrieval process and reduce the amount of unnecessary data transferred between the server and the client. This can improve the overall performance and loading times of your application.
- Modularity: Custom APIs promote modularity in your Nuxt.js project. You can create separate API modules for different sections of your application, making it easier to manage and maintain your codebase.
Steps to Develop Custom APIs
Developing custom APIs in Nuxt.js involves the following steps:
- Defining API Endpoints: Identify the data you need to fetch and determine the API endpoints required to retrieve that data. These endpoints can be defined using the Nuxt.js serverMiddleware configuration.
- Implementing API Logic: Write the logic to handle the API requests and responses. This includes fetching data from external sources, performing any necessary data transformations or calculations, and returning the data in the desired format.
- Testing and Validation: Thoroughly test the custom APIs to ensure they function as expected. Validate the data returned by the APIs and handle any potential errors or edge cases.
- Documenting the APIs: Document your custom APIs, including the available endpoints, expected request parameters, and response formats. This documentation will be useful for both yourself and any other developers working on the project.
By following these steps, you can create powerful custom APIs that seamlessly integrate with your Nuxt.js project. Remember to test your APIs thoroughly and ensure they adhere to best practices for security and performance. For more information on Nuxt.js custom development, check out our article on nuxt.js custom development.
Creating custom APIs in Nuxt.js opens up a world of possibilities for your application. It allows you to tailor the data flow to your specific needs and leverage external resources to enhance the functionality of your project. With the right approach and careful planning, custom APIs can be a valuable asset in your Nuxt.js development journey.
Integrating Custom APIs in Your Nuxt.js Project
Once you have developed your custom APIs in Nuxt.js, the next step is to seamlessly integrate them into your project. This involves connecting the APIs to your frontend and handling the responses effectively.
Connecting APIs to Your Frontend
To connect your custom APIs to the frontend of your Nuxt.js project, you will need to make use of the axios
module. Nuxt.js provides built-in support for Axios, a popular HTTP client library. This makes it easier to send requests to your APIs and retrieve the data needed for your application.
To get started, you will need to install the Axios module by running the following command in your Nuxt.js project:
npm install @nuxtjs/axios
After installing the module, you can configure it in your nuxt.config.js
file. Here’s an example of how to set it up:
// nuxt.config.js export default { modules: [ '@nuxtjs/axios', ], axios: { // API endpoint configuration baseURL: 'https://api.example.com', // Additional axios options // ... }, }
With the Axios module set up, you can now make HTTP requests to your custom APIs using the this.$axios
instance in your Vue components. For example, to fetch data from a custom API endpoint, you can use the get
method like this:
export default { async mounted() { const response = await this.$axios.get('/api/data') // Handle the API response here } }
Handling API Responses
When integrating custom APIs in your Nuxt.js project, it’s important to handle the API responses effectively. This includes handling errors, processing the received data, and updating the frontend accordingly.
For successful API responses, you can access the data returned by the API using the response.data
property. You can then use this data to update your Vue component’s state or render it in the template.
In case of errors, you can utilize error handling mechanisms provided by Axios. For example, you can use a try-catch
block to handle errors and display appropriate error messages to the user. Here’s an example:
export default { methods: { async fetchData() { try { const response = await this.$axios.get('/api/data') // Handle the successful response here } catch (error) { // Handle the error here } } } }
Additionally, you can take advantage of Nuxt.js error handling features to handle errors globally. This allows you to define a layout component specifically for error pages, providing a consistent user experience when API requests encounter errors.
By effectively connecting your custom APIs to the frontend using the Axios module and handling the API responses appropriately, you can ensure that your Nuxt.js project seamlessly interacts with your backend and provides a smooth user experience.
In the next section, we will explore the importance of testing custom APIs in Nuxt.js and discuss various debugging techniques.
Testing and Debugging
When developing custom APIs in Nuxt.js, testing and debugging are crucial steps to ensure the reliability and functionality of your APIs. By thoroughly testing your APIs and employing effective debugging techniques, you can identify and resolve any issues or errors that may arise during development. This section will highlight the importance of testing custom APIs and provide insights into debugging techniques for a smooth development process.
Importance of Testing Custom APIs
Testing custom APIs is essential to verify that they are functioning as intended. Here are a few reasons why testing custom APIs in Nuxt.js is of utmost importance:
- Functionality Validation: Testing allows you to validate the functionality of your custom APIs. By running various test cases, you can ensure that your APIs perform the intended operations correctly, handle different inputs, and provide accurate responses.
- Error Detection and Handling: Testing helps identify any errors or bugs in your custom APIs. By systematically testing different scenarios, you can pinpoint issues in the code, such as incorrect data manipulation, logic errors, or unexpected behavior. This allows you to address these issues and improve the overall reliability of your APIs.
- Integration Testing: Integration testing involves testing the interaction between different components of your application. It ensures that your custom APIs seamlessly integrate with other components, such as the frontend or external services, without any compatibility or communication issues.
- Performance Evaluation: Testing custom APIs helps evaluate their performance under different loads and conditions. This includes checking response times, handling concurrent requests, and assessing scalability. By conducting performance testing, you can optimize your APIs to deliver fast and efficient responses.
To facilitate effective testing, you can use testing frameworks like Jest or Mocha, which provide tools and utilities specifically designed for testing in the Nuxt.js ecosystem.
Debugging Techniques for Custom APIs
Debugging is an essential part of the development process that involves identifying and resolving issues in your custom APIs. Here are some techniques to help you debug your custom APIs effectively:
- Logging: Incorporate logging statements strategically throughout your code to track the flow of data and identify potential issues. By logging relevant information, such as input parameters, intermediate results, and error messages, you can gain insights into the execution flow and pinpoint areas that require attention.
- Error Handling: Proper error handling is critical for effective debugging. Implement robust error handling mechanisms within your custom APIs, including try-catch blocks, to catch and handle exceptions gracefully. By logging or displaying meaningful error messages, you can quickly identify and address issues during development.
- Debugging Tools: Take advantage of debugging tools provided by Nuxt.js and its associated plugins. These tools enable you to set breakpoints, step through code execution, inspect variables, and analyze the runtime behavior of your custom APIs. Utilizing a debugger can significantly streamline the debugging process and aid in identifying the root cause of issues.
- Testing with Mock Data: Use mock data during testing to simulate different scenarios and edge cases. By providing specific inputs and observing the corresponding outputs, you can validate the behavior of your custom APIs and identify potential issues. Mock data allows you to isolate and test specific functionalities without relying on external dependencies.
By combining effective testing practices with efficient debugging techniques, you can ensure the quality and reliability of your custom APIs in Nuxt.js. Regular testing and debugging throughout the development process help you catch and resolve issues early, resulting in a robust and stable API that meets the needs of your application.
Advanced Features and Best Practices
Once you have developed your custom APIs in Nuxt.js, there are advanced features and best practices that you can implement to enhance the performance, caching, and security of your application.
Caching and Performance Optimization
Caching is an essential aspect of optimizing the performance of your Nuxt.js custom APIs. By implementing caching techniques, you can reduce the load on your server and improve the response time of your APIs. Here are some caching strategies you can consider:
- Client-Side Caching: Utilize browser caching mechanisms such as HTTP caching headers (e.g.,
Cache-Control
,Expires
) to enable client-side caching of API responses. This allows the client to store and reuse the response for subsequent requests, reducing the need for unnecessary server calls. - Server-Side Caching: Implement server-side caching mechanisms such as in-memory caching or a distributed caching system. This can be achieved using tools like Redis or Memcached. By caching frequently accessed data or API responses, you can significantly improve the response time and reduce the load on your server.
- Content Delivery Networks (CDNs): Consider leveraging CDNs to cache static assets and API responses. CDNs store copies of your content in multiple geographic locations, reducing the latency for users across different regions.
- Optimized Database Queries: Analyze and optimize your database queries to ensure efficient retrieval and manipulation of data. Proper indexing, query optimization techniques, and avoiding unnecessary database calls can help improve the overall performance of your custom APIs.
Remember to regularly monitor the performance of your APIs using appropriate tools and profiling techniques. This will help you identify any bottlenecks and make necessary optimizations to ensure a smooth user experience.
Security Measures for Custom APIs
When developing custom APIs in Nuxt.js, it’s crucial to prioritize the security of your application and protect sensitive user data. Here are some best practices for securing your custom APIs:
- Authentication and Authorization: Implement robust authentication mechanisms, such as JWT (JSON Web Tokens) or OAuth, to ensure that only authorized users can access your APIs. Validate user credentials and assign appropriate access levels to control what actions they can perform.
- Input Validation and Sanitization: Validate and sanitize user input to prevent common security vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Utilize libraries and frameworks that provide built-in validation and sanitization features.
- Secure Communication: Encrypt communication between your client and server using HTTPS (HTTP over SSL/TLS). This ensures that sensitive data transmitted over the network remains secure and protected from eavesdropping or tampering.
- Rate Limiting: Implement rate limiting mechanisms to prevent abuse and protect your APIs from malicious attacks or excessive usage. Rate limiting controls the number of requests a client can make within a specified time frame, preventing potential misuse or denial-of-service attacks.
- Error Handling and Logging: Implement proper error handling and logging mechanisms to capture any unexpected exceptions or errors that may occur during API execution. This helps in identifying and resolving potential security issues or vulnerabilities.
Regularly update your dependencies and libraries to ensure that you are using the latest versions, which often include security patches and bug fixes. Stay informed about common security practices and emerging threats to keep your custom APIs protected.
By implementing caching and performance optimization techniques, as well as following security best practices, you can ensure that your Nuxt.js custom APIs are efficient, reliable, and secure.