Nuxt.js Custom Component Development Essentials
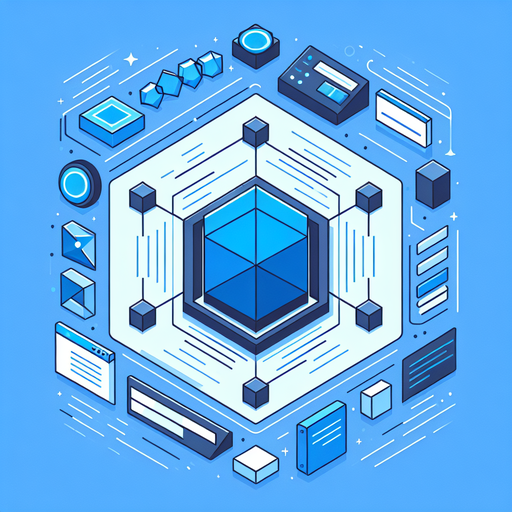
Contenidos
Introduction to Nuxt.js Custom Component Development
In the world of web development, building dynamic and interactive user interfaces is essential. Nuxt.js, a powerful framework based on Vue.js, provides a robust foundation for creating exceptional web applications. One of the key aspects of Nuxt.js development is custom component development, which allows developers to create reusable and modular UI elements.
Importance of Custom Components in Nuxt.js
Custom components play a vital role in Nuxt.js development. They enable developers to break down complex user interfaces into smaller, manageable pieces. By encapsulating specific functionalities and styles within custom components, developers can reuse these components across different pages and projects, saving time and effort.
The use of custom components promotes code reusability and maintainability. Instead of writing redundant code for similar UI elements, developers can create custom components that encapsulate the necessary logic and styling. This not only reduces code duplication but also ensures consistency throughout the application.
Furthermore, custom components enhance the scalability of Nuxt.js applications. As the project grows, the ability to reuse components becomes invaluable. Developers can build upon existing custom components, adding new features and functionalities without reinventing the wheel. This modular approach simplifies the development process and allows for faster iteration.
Overview of Custom Component Development Essentials
To develop custom components in Nuxt.js, it’s essential to have a good understanding of Vue.js and its component-based architecture. Vue.js provides a declarative syntax for building UI components, making it intuitive and efficient.
In custom component development, developers need to grasp concepts such as props, which allow data to be passed from parent components to child components. Additionally, knowledge of computed properties, watchers, and lifecycle hooks is beneficial for creating dynamic and interactive custom components.
Understanding the structure of Nuxt.js projects and the Vue.js single-file component format is also crucial. This includes knowledge of the <template>
, <script>
, and <style>
sections within a single file component, allowing developers to define the HTML, JavaScript, and CSS aspects of a custom component in a concise and organized manner.
By mastering these custom component development essentials, developers can harness the full potential of Nuxt.js and create highly customizable and reusable UI elements. In the following sections, we will explore various aspects of custom component development, including styling, enhancing functionality, and testing.
Setting Up Your Development Environment
Before diving into custom component development in Nuxt.js, it’s important to set up your development environment properly. This ensures that you have all the necessary tools and configurations in place to successfully create and test your custom components. In this section, we will cover the steps to install Nuxt.js and configure your project for custom component development.
Installing Nuxt.js
To begin, you’ll need to install Nuxt.js, a powerful framework for building Vue.js applications. Nuxt.js provides a structured and optimized development environment, making it an ideal choice for custom component development. Here’s how you can install Nuxt.js:
- Open your terminal or command prompt.
- Navigate to your project directory.
- Run the following command to install Nuxt.js globally:
npm install -g create-nuxt-app
This command installs the create-nuxt-app
package, which allows you to quickly set up a new Nuxt.js project.
- Once the installation is complete, run the following command to create a new Nuxt.js project:
npx create-nuxt-app <project-name>
Replace <project-name>
with the desired name for your project.
- Follow the prompts to configure your project. You can choose options such as the package manager (npm or yarn), the UI framework, the testing framework, and more.
After completing these steps, Nuxt.js will be installed in your project and you’ll have a basic project structure ready for custom component development.
Configuring Your Project for Custom Component Development
Now that you have Nuxt.js installed, it’s time to configure your project specifically for custom component development. This involves setting up the necessary files and configurations to ensure a smooth development process. Here are the key steps to configure your project:
- Open your project directory in your preferred code editor.
- Locate the
pages
directory within your project structure. This directory contains the pages of your Nuxt.js application. - Create a new directory within the
pages
directory calledcomponents
. This directory will house your custom components. - Within the
components
directory, you can create individual Vue component files for each custom component you plan to develop. For example, you could create a file calledMyCustomComponent.vue
to define your first custom component. - Begin writing the code for your custom components in the respective Vue component files. You can define the structure, behavior, and styling of your components using Vue.js syntax and techniques.
By following these steps, you have successfully set up your development environment for custom component development in Nuxt.js. You can now start creating and integrating your custom components into your Nuxt.js application. Remember to refer to the Nuxt.js documentation and utilize available resources for further guidance on building custom components with Nuxt.js.
Creating Custom Components in Nuxt.js
Custom components play a vital role in Nuxt.js development, allowing you to build interactive and reusable elements for your applications. In this section, we will explore the fundamentals of creating custom components in Nuxt.js, including understanding Vue components and writing custom components specific to Nuxt.js.
Understanding Vue Components
To create custom components in Nuxt.js, it’s important to have a good understanding of Vue components. Vue components are the building blocks of a Vue application, encapsulating the HTML, CSS, and JavaScript logic required to render a specific part of the user interface.
A Vue component consists of three main parts:
- Template: The HTML structure that defines the appearance of the component.
- Script: The JavaScript code that handles the component’s behavior and data.
- Style: The CSS styling that defines the component’s visual presentation.
Vue components promote reusability and modularity, enabling you to create complex applications by combining and nesting multiple components. In Nuxt.js, you can take advantage of Vue’s component-based architecture to create custom components that fit seamlessly into your application’s structure.
Writing Custom Components in Nuxt.js
In Nuxt.js, you can create custom components by following a simple directory structure. By default, Nuxt.js uses the components
directory to store your custom components. Inside this directory, you can organize your components by creating separate subdirectories for different components or component categories.
To create a custom component in Nuxt.js, follow these steps:
- Create a new
.vue
file inside thecomponents
directory or its subdirectory. - Define the HTML structure of the component using the
<template>
tag. - Add the JavaScript logic for the component inside the
<script>
tag. - Style the component using CSS inside the
<style>
tag.
Here’s an example of a basic custom component in Nuxt.js:
<template> <div> <h1>{{ message }}</h1> </div> </template> <script> export default { data() { return { message: 'Hello, world!' } } } </script> <style scoped> h1 { color: blue; } </style>
Once you have created a custom component, you can use it in your Nuxt.js application by simply importing and registering it in the desired page or layout file. This allows you to reuse the component across different pages, enhancing code modularity and maintainability.
By understanding Vue components and following the Nuxt.js conventions for creating custom components, you can unleash the full potential of Nuxt.js in developing powerful and interactive applications. Make sure to also explore other aspects of Nuxt.js custom development, such as Nuxt.js custom theme development and Nuxt.js custom plugin development, to further enhance your application’s capabilities.
Styling and Theming Custom Components
When it comes to developing custom components in Nuxt.js, styling and theming play a crucial role in creating visually appealing and cohesive user interfaces. In this section, we will explore two essential aspects of styling and theming custom components: utilizing CSS frameworks and implementing custom styles.
Utilizing CSS Frameworks
CSS frameworks provide a collection of pre-designed CSS rules and components that can be easily integrated into your Nuxt.js project. These frameworks offer a solid foundation for styling your custom components, saving you time and effort in writing CSS from scratch.
By leveraging CSS frameworks such as Bootstrap, Tailwind CSS, or Bulma, you can take advantage of their responsive grids, typography, and ready-to-use UI components. These frameworks are designed to be highly customizable, allowing you to tailor the styles to match your specific project requirements.
To integrate a CSS framework into your Nuxt.js project, you can either install it via npm or include it via a CDN link. Once installed or linked, you can start incorporating the framework’s classes and components into your custom components, making styling a breeze.
Implementing Custom Styles for Components
While CSS frameworks provide a great starting point, you may also need to implement custom styles to achieve the desired look and feel for your custom components. Nuxt.js offers flexibility in terms of styling options, allowing you to utilize CSS modules, inline styles, or even CSS preprocessors like Sass or Less.
CSS modules enable you to scope CSS styles to specific components, preventing clashes with other styles in your project. This helps to maintain a modular and maintainable codebase. By using CSS modules, you can import and apply styles directly to your custom components, ensuring encapsulation and reusability.
For more advanced styling needs, you can leverage CSS preprocessors like Sass or Less. These preprocessors provide additional features such as variables, mixins, and nesting, allowing for more efficient and organized stylesheets. Nuxt.js seamlessly integrates with CSS preprocessors, making it easy to implement custom styles in your custom components.
When styling your custom components, it’s important to follow best practices and adhere to a consistent design system. This ensures a cohesive and professional user interface across your application. Consider creating a style guide or design system to document your project’s visual guidelines, including color schemes, typography, and component styles.
By utilizing CSS frameworks and implementing custom styles, you can create visually appealing and well-designed custom components in your Nuxt.js project. Remember to leverage the power of CSS modules, preprocessors, and a consistent design system to streamline your styling process. For more information on Nuxt.js custom development, check out our article on nuxt.js custom development.
Enhancing Functionality with Custom Components
To take your Nuxt.js application to the next level, enhancing functionality with custom components is key. This section will explore two essential aspects of custom component development: implementing interactivity and integrating third-party libraries.
Implementing Interactivity
Interactivity is vital for creating engaging and dynamic user experiences. In Nuxt.js, you can implement interactivity in your custom components using various techniques. Here are a few approaches you can take:
- Event Handling: Utilize Vue’s event handling system to capture user actions and trigger specific behaviors within your custom components. This allows you to create interactive elements such as buttons, forms, and navigation menus.
- Data Binding: Leverage Vue’s two-way data binding to establish a connection between the component’s data and the user interface. This enables real-time updates and synchronization between the user input and the component’s state.
- Computed Properties: Take advantage of computed properties in Vue to perform dynamic calculations or transformations based on the component’s data. Computed properties are ideal for updating the component’s output based on user input or other data changes.
By implementing interactivity in your custom components, you can create more engaging and responsive user interfaces.
Integrating Third-Party Libraries
Integrating third-party libraries can greatly expand the functionality of your custom components in Nuxt.js. These libraries provide pre-built solutions for various tasks, saving you development time and effort. Here are some key points to consider when integrating third-party libraries:
- Research and Evaluation: Before integrating a library, thoroughly research and evaluate its features, documentation, community support, and compatibility with Nuxt.js. Choose libraries that align with your project requirements and have a proven track record of stability and reliability.
- Installation and Configuration: Follow the library’s installation instructions, which typically involve installing the library using npm or yarn. Then, configure the library in your Nuxt.js project, ensuring that it is properly imported and accessible within your custom components.
- Component Integration: Once the library is installed and configured, leverage its components, functions, or utilities within your custom components. This allows you to leverage the library’s features seamlessly and enhance the functionality of your application.
- Maintaining Compatibility: Keep an eye on updates and releases for the integrated libraries. Ensure that your custom components remain compatible by updating the libraries to their latest versions. This helps to ensure smooth operation and access to any bug fixes or new features.
By integrating third-party libraries into your custom components, you can leverage existing solutions and tap into a wide range of functionalities. However, always exercise caution and thoroughly test the integration to ensure compatibility and avoid any conflicts with your Nuxt.js project.
Incorporating interactivity and integrating third-party libraries are essential steps in developing powerful and feature-rich custom components in Nuxt.js. By implementing these techniques, you can enhance the functionality of your application and create a compelling user experience.
Testing and Debugging Custom Components
When developing custom components in Nuxt.js, testing and debugging play a crucial role in ensuring the functionality and quality of your components. In this section, we will explore the importance of testing custom components and provide some debugging techniques to help you identify and fix any issues that may arise.
Importance of Testing Custom Components
Testing your custom components is essential to ensure that they behave as expected and deliver the desired functionality. Proper testing helps identify any bugs or errors early in the development process, allowing you to address them before your components are deployed.
By testing your custom components, you can verify that they integrate seamlessly with other parts of your application and interact correctly with data sources and APIs. It also helps ensure that your components respond appropriately to user input and handle edge cases gracefully.
Testing custom components can be done using various approaches, such as unit testing, integration testing, and end-to-end testing. Each approach serves a different purpose and helps you validate different aspects of your components. Incorporating a robust testing strategy into your development workflow significantly reduces the risk of introducing regressions and enhances the overall stability of your application.
Debugging Techniques for Custom Components
Despite thorough testing, it’s not uncommon to encounter issues or unexpected behavior in your custom components. When this happens, effective debugging techniques can help you identify and resolve the underlying problems.
One approach to debugging custom components is to use browser developer tools. These tools provide a range of features, including console logging, inspecting DOM elements, and monitoring network requests. By examining console logs and inspecting the state of your components, you can gain insights into the execution flow and pinpoint potential issues.
Another useful technique is to leverage Vue.js Devtools, a browser extension that enhances the debugging experience for Vue.js applications. With Vue.js Devtools, you can inspect the component hierarchy, examine component props and data, and even manipulate the state in real-time. This powerful tool can help you identify and diagnose issues more efficiently.
Apart from browser-based debugging, incorporating automated error tracking and logging tools into your application can provide valuable insights into the errors occurring in your custom components. These tools can capture and report exceptions, stack traces, and other critical information, allowing you to quickly identify the root cause of the problem.
Furthermore, writing clear and comprehensive error messages within your custom components can aid in debugging. Well-crafted error messages provide valuable context and guide developers in understanding the cause of errors, making it easier to fix them.
Remember, the key to effective debugging is to approach it systematically. Start by reproducing the issue consistently and isolating the problematic component or code section. Then, use the available debugging tools and techniques to dive deep into the execution flow and identify the source of the problem. For complex issues, consider reaching out to the Nuxt.js community or referring to relevant documentation for additional guidance.
By prioritizing testing and employing effective debugging techniques, you can ensure the quality and reliability of your custom components in Nuxt.js. This allows you to deliver a seamless and error-free user experience while building your application with confidence.