Exploring Laravel Custom API Development
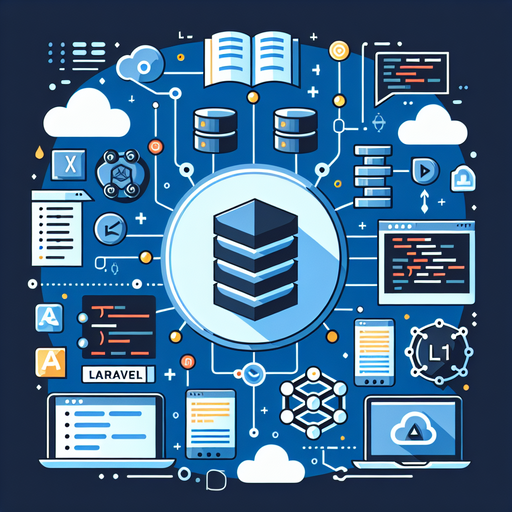
Contenidos
Understanding Laravel Custom API Development
Laravel custom API development is a powerful approach that allows businesses to create tailored APIs using the Laravel framework. These APIs serve as the backbone for communication between different software systems, enabling seamless integration and data exchange. In this section, we will explore the introduction to Laravel custom API development and the benefits it offers to businesses.
Introduction to Laravel Custom API Development
Laravel custom API development is the process of designing and building APIs specifically tailored to meet the unique needs of a business. Laravel, a popular PHP framework, provides a robust foundation for developing APIs that are scalable, secure, and efficient. With Laravel’s expressive syntax and extensive feature set, developers can create custom APIs that align perfectly with the business requirements.
Laravel custom APIs act as intermediaries, facilitating communication between various software components, applications, or even third-party services. They enable seamless data exchange and integration, allowing businesses to streamline workflows, automate processes, and enhance overall productivity.
Benefits of Custom API Development with Laravel
Custom API development with Laravel offers numerous benefits to businesses. Some of the key advantages include:
- Flexibility: Laravel provides a flexible environment for developing custom APIs. Developers have the freedom to design APIs that align with specific business needs, allowing for efficient data exchange and integration.
- Scalability: Laravel’s modular architecture and robust ecosystem make it easy to scale custom APIs as the business grows. Whether it’s handling increased traffic or accommodating new features, Laravel APIs can be easily expanded to meet evolving business requirements.
- Security: Laravel comes with built-in security features that help developers ensure the safety of custom APIs. From authentication and authorization mechanisms to data encryption, Laravel provides a solid foundation for implementing secure APIs.
- Efficiency: Laravel’s expressive syntax and rich set of features enable developers to build APIs quickly and efficiently. This reduces development time and effort, allowing businesses to bring their APIs to market faster and gain a competitive edge.
- Integration: Custom APIs developed with Laravel can seamlessly integrate with existing business systems, such as CRM software, project management tools, or e-commerce platforms. This integration enables real-time data synchronization and enhances overall operational efficiency.
- Compatibility: Laravel APIs can be developed to comply with industry standards and best practices, ensuring compatibility with other systems and services. This compatibility facilitates collaboration and data exchange with partners, vendors, or clients.
By leveraging Laravel custom API development, businesses can revolutionize their operations, enhance productivity, and unlock new opportunities for growth. To learn more about Laravel development and its potential, check out our article on how-to-develop-scalable-laravel-apps.
In the next sections, we will explore the fundamental aspects of Laravel custom API development, including the Laravel framework itself and the importance of APIs in Laravel development. Stay tuned!
Laravel Basics
To understand the concept of Laravel custom API development, it’s essential to have a solid grasp of the Laravel framework and the role of APIs within it.
Overview of Laravel Framework
Laravel is a popular open-source PHP framework known for its elegance, simplicity, and robust features. It provides developers with a structured and efficient way to build web applications. Laravel follows the Model-View-Controller (MVC) architectural pattern, making it easier to separate the business logic from the presentation layer.
Some key features of Laravel include:
- Routing: Laravel’s routing system allows developers to define clean and intuitive URLs for their web applications. By mapping URLs to controller actions, developers can handle incoming requests effectively.
- Database Integration: Laravel offers a powerful ORM (Object-Relational Mapping) called Eloquent, which simplifies database operations. It supports various database systems, including MySQL, PostgreSQL, SQLite, and SQL Server.
- Authentication and Authorization: Laravel provides an easy-to-use authentication system out of the box. Developers can quickly implement user registration, login, and password reset functionality. Additionally, Laravel’s authorization features allow fine-grained control over user permissions and roles.
For a more comprehensive understanding of Laravel, you can refer to our article on overview-laravel-custom-development.
Importance of APIs in Laravel Development
APIs (Application Programming Interfaces) play a vital role in Laravel development, enabling seamless communication between different systems and services. APIs act as intermediaries, allowing applications to interact with each other and share data in a structured manner.
In Laravel, APIs can be used to expose functionalities and resources of a web application to other applications or clients. This allows for the development of custom APIs that cater to specific business needs. Laravel’s built-in API features, such as API routes and resource controllers, make it easier to create and manage APIs.
Here are some key benefits of using APIs in Laravel development:
- Integration: APIs enable integration between different systems, applications, and services. By exposing specific endpoints, developers can facilitate data exchange and functionality sharing, leading to enhanced collaboration and efficiency.
- Scalability: APIs provide a scalable approach to application development. By decoupling the frontend and backend, developers can make changes and updates without affecting the entire system. This modular approach allows for more flexibility and easier maintenance.
- Customization: Custom APIs built with Laravel allow businesses to tailor their application’s functionalities to their specific needs. Whether it’s retrieving data, performing calculations, or triggering actions, APIs provide the ability to develop custom solutions that align with business requirements.
For more insights into Laravel custom development and best practices, you can refer to our article on how-to-develop-scalable-laravel-apps.
Understanding the basics of Laravel and the significance of APIs in Laravel development sets the foundation for exploring the world of Laravel custom API development. In the next sections, we will delve into the planning, designing, and building of custom APIs using Laravel, as well as considerations for integration, testing, security, and performance optimization.
Developing Custom APIs with Laravel
To harness the power of Laravel for custom API development, it is essential to understand the process of planning, designing, and building APIs. This section will explore the key steps involved in developing custom APIs with Laravel.
Planning and Designing APIs
Before diving into the development process, careful planning and designing of APIs are crucial. This phase sets the foundation for a successful API implementation. Here are some key considerations during the planning and designing phase:
- Identify API Use Cases: Determine the specific use cases for your API. Understand the requirements of your business and how the API will serve those needs. Consider the functionalities, endpoints, and data that your API will expose.
- API Design Principles: Following RESTful design principles can simplify the development and usage of your API. Design clear and intuitive endpoints, utilize appropriate HTTP methods, and ensure consistency in response formats.
- Data Structure and Validation: Define the structure and format of the data that will be exchanged through the API. Implement data validation to ensure that the incoming requests meet the required specifications.
- Authentication and Authorization: Determine the authentication and authorization mechanisms to secure your API. Laravel provides various authentication methods like API tokens, OAuth, and JWT (JSON Web Tokens) to protect your APIs from unauthorized access.
Building APIs with Laravel
Once the planning and designing phase is complete, it’s time to start building your custom APIs with Laravel. Laravel provides a robust framework that simplifies API development. Here are the key steps involved in building APIs with Laravel:
- Routing: Utilize Laravel’s powerful routing system to define the API endpoints and map them to specific controller actions. Laravel’s expressive syntax makes it easy to handle different HTTP methods and route parameters.
- Controllers and Models: Create controllers that handle the business logic for each API endpoint. Leverage Laravel’s model-view-controller (MVC) architecture to separate concerns and maintain a clean and organized codebase.
- Request Handling and Validation: Laravel provides convenient methods for handling incoming requests and validating the data. Use Laravel’s request classes to handle the request inputs and validate them against defined rules.
- Response Formatting: Format and structure the API responses to follow consistent standards. Laravel’s built-in response methods allow you to return responses in various formats like JSON, XML, or even custom formats.
- Error Handling: Implement error handling mechanisms to provide informative and meaningful error responses when API requests encounter issues. Laravel’s exception handling system simplifies error reporting and handling.
- API Documentation: Document your APIs to provide clear instructions and guidelines for developers who will be consuming your API. Tools like Laravel’s Swagger integration or external solutions like Postman can assist in generating API documentation.
By following these steps, you can effectively develop custom APIs using the Laravel framework. Remember to leverage the vast Laravel ecosystem, which includes various packages and libraries, to enhance and streamline your API development process. For more insights into developing scalable Laravel applications, check out our article on how to develop scalable Laravel apps.
In the next section, we will explore the integration and testing phase of custom API development with Laravel. Stay tuned!
Integration and Testing
As you embark on your Laravel custom API development journey, it’s essential to understand the process of integrating APIs into your business systems and the importance of testing and debugging custom APIs to ensure their functionality.
Integrating APIs into Your Business Systems
Integrating APIs into your business systems is a crucial step in leveraging the power of Laravel custom API development. APIs serve as the bridge between different software applications, allowing them to communicate and share data seamlessly. By integrating APIs into your business systems, you can streamline processes, enhance efficiency, and improve overall productivity.
To integrate APIs effectively, it’s important to have a clear understanding of your business requirements and the specific functionalities you want to achieve. This involves identifying the APIs that align with your needs and ensuring they are compatible with your existing systems. Laravel provides a robust framework for API integration, offering a wide range of libraries and tools to facilitate seamless integration with various platforms and technologies.
During the integration process, it’s essential to consider factors such as security, data synchronization, and error handling. By following best practices and utilizing Laravel’s built-in features, you can ensure a smooth and secure integration of APIs into your business systems. For more information on Laravel development and project management, check out our articles on how to develop scalable Laravel apps and Laravel project management.
Testing and Debugging Custom APIs
Testing and debugging are critical steps in the development of custom APIs with Laravel. Thorough testing ensures that your APIs function as intended, provide accurate responses, and handle errors gracefully. By identifying and fixing any issues during the testing and debugging phase, you can ensure the reliability and stability of your custom APIs.
To effectively test and debug custom APIs, it’s important to have a well-defined testing strategy in place. This includes creating comprehensive test cases that cover different scenarios and edge cases. Laravel provides a testing framework that simplifies the process of writing and executing tests, allowing you to validate the functionality and performance of your APIs.
During the testing phase, it’s important to validate the input and output of your APIs, test various endpoints, and simulate different user interactions. This helps uncover any potential bugs, performance bottlenecks, or security vulnerabilities. By utilizing Laravel’s debugging tools and error handling mechanisms, you can identify and resolve issues efficiently.
Remember to document any issues and fixes encountered during the testing and debugging process. This documentation serves as a valuable resource for future reference and maintenance of your custom APIs.
Integrating APIs into your business systems and thoroughly testing and debugging your custom APIs are crucial steps to ensure the successful implementation of Laravel custom API development. By following best practices and leveraging the power of Laravel’s features and tools, you can create robust and reliable APIs that meet your business needs. For more insights on Laravel custom development, explore our articles on overview of Laravel custom development and Laravel custom development in Barcelona.
Security and Performance Considerations
When it comes to Laravel custom API development, ensuring the security and optimizing the performance of your APIs are critical aspects to consider. In this section, we will explore the steps you can take to ensure the security of your custom APIs and optimize their performance.
Ensuring Security in Custom APIs
Security is of utmost importance when developing custom APIs with Laravel. Here are some key considerations to keep in mind to ensure the security of your APIs:
- Authentication and Authorization: Implement a robust authentication and authorization mechanism to ensure that only authorized users or applications can access your APIs. Laravel provides various authentication methods, such as token-based authentication or OAuth, which can be tailored to your specific requirements.
- Input Validation and Sanitization: Validate and sanitize all user inputs to prevent common security vulnerabilities, such as SQL injection and cross-site scripting (XSS) attacks. Laravel offers built-in validation and sanitization features that can be easily implemented to ensure the integrity of your API data.
- Data Encryption: Protect sensitive data by encrypting it during transmission and storage. Laravel provides encryption mechanisms that enable you to secure sensitive information, such as user credentials or confidential data, effectively.
- Rate Limiting: Implement rate limiting to prevent abuse of your APIs and mitigate the risk of denial-of-service (DoS) attacks. By setting limits on the number of requests per minute or hour, you can control the usage of your APIs and protect them from potential malicious activities.
- Error Handling and Logging: Proper error handling and logging are essential for identifying and addressing security issues. Laravel’s error handling and logging capabilities allow you to capture and monitor errors, exceptions, and other security-related events, enabling you to quickly respond to any potential threats.
Optimizing Performance of Laravel Custom APIs
To ensure optimal performance of your Laravel custom APIs, consider the following strategies:
- Caching: Utilize caching mechanisms, such as Laravel’s built-in caching system or external caching solutions like Redis, to store frequently accessed data. This reduces the need for repeated database queries, improving the response time of your APIs.
- Query Optimization: Optimize your database queries to minimize the time required to fetch or manipulate data. Use Laravel’s query builder or Eloquent ORM to write efficient and optimized queries that retrieve only the necessary data.
- Code Optimization: Identify and refactor any inefficient code that may impact the performance of your APIs. Optimize resource-intensive operations, reduce redundant code, and leverage Laravel’s features, such as eager loading and lazy loading, to optimize the execution of your API code.
- Load Balancing and Scaling: Implement load balancing techniques and scale horizontally by distributing incoming API requests across multiple servers. This helps to distribute the workload and maintain the performance of your APIs, even during periods of high traffic.
- Monitoring and Profiling: Continuously monitor and profile your APIs to identify performance bottlenecks. Laravel provides tools like Telescope that allow you to track and analyze API performance, database queries, and other vital metrics. By monitoring and profiling your APIs, you can identify areas that require optimization and fine-tuning.
By prioritizing security and performance in your Laravel custom API development, you can build robust and efficient APIs that meet the needs of your business and ensure a seamless experience for your users. Stay updated with the latest trends and best practices in Laravel custom development to leverage the full potential of this powerful framework.
Future Trends in Laravel Custom API Development
As technology continues to evolve, the field of Laravel custom API development is also advancing at a rapid pace. Staying up-to-date with emerging technologies and practices is essential for businesses looking to revolutionize their operations. In this section, we will explore some of the future trends in Laravel custom API development and how they can be leveraged for business growth.
Emerging Technologies and Practices
Microservices Architecture
Microservices architecture is gaining popularity in Laravel custom API development. It involves breaking down complex applications into smaller, independent services that can be developed, deployed, and scaled individually. This approach allows for greater flexibility, scalability, and maintainability of the APIs. By adopting microservices architecture, businesses can build modular and highly scalable systems that can adapt to changing requirements.
GraphQL
GraphQL is a query language for APIs that provides a more efficient and flexible alternative to traditional RESTful APIs. It allows clients to specify exactly what data they need from the API, reducing over-fetching and under-fetching of data. Laravel has excellent support for GraphQL through packages like Lighthouse, enabling developers to build powerful and performant APIs. Embracing GraphQL can enhance the developer experience and improve the overall performance of your Laravel custom APIs.
Serverless Architecture
Serverless architecture is gaining traction in the development community. It allows developers to build and run applications without the need to manage servers or infrastructure. With serverless computing platforms like AWS Lambda and Azure Functions, developers can focus on writing code and let the platform handle the scaling and management of resources. Adopting serverless architecture in Laravel custom API development can bring cost savings, scalability, and reduced operational overhead.
Leveraging Custom APIs for Business Growth
Custom APIs developed with Laravel offer immense opportunities for businesses to drive growth and innovation. Here are some ways to leverage custom APIs for business growth:
Integration with Third-Party Services
Integrating custom APIs with third-party services can expand the capabilities of your business systems. By connecting your Laravel application with popular applications and services, you can streamline workflows, automate tasks, and enhance user experiences. Whether it’s integrating with payment gateways, social media platforms, or CRM systems, custom APIs provide the flexibility to create seamless and efficient integrations.
Building API Ecosystems
Building an API ecosystem allows businesses to expose their services to external developers and partners. By providing well-documented APIs and developer resources, businesses can foster innovation and collaboration. This can lead to the creation of new applications, integrations, and services that leverage your core offerings. A thriving API ecosystem can drive business growth by expanding your reach and creating new revenue streams.
Mobile Application Development
Custom APIs play a pivotal role in mobile application development. Leveraging Laravel custom APIs, businesses can develop mobile applications that seamlessly integrate with their existing systems. This enables enhanced customer experiences, real-time updates, and access to critical business data on the go. By investing in mobile application development and utilizing custom APIs, businesses can tap into the growing mobile market and stay ahead of the competition.
As Laravel custom API development continues to evolve, businesses must adapt to emerging technologies and practices to stay relevant. By embracing trends like microservices architecture, GraphQL, and serverless computing, businesses can build scalable, performant, and innovative systems. Leveraging custom APIs for integration, building ecosystems, and mobile application development can drive business growth and unlock new opportunities in the digital landscape.