How to develop a WordPress plugin
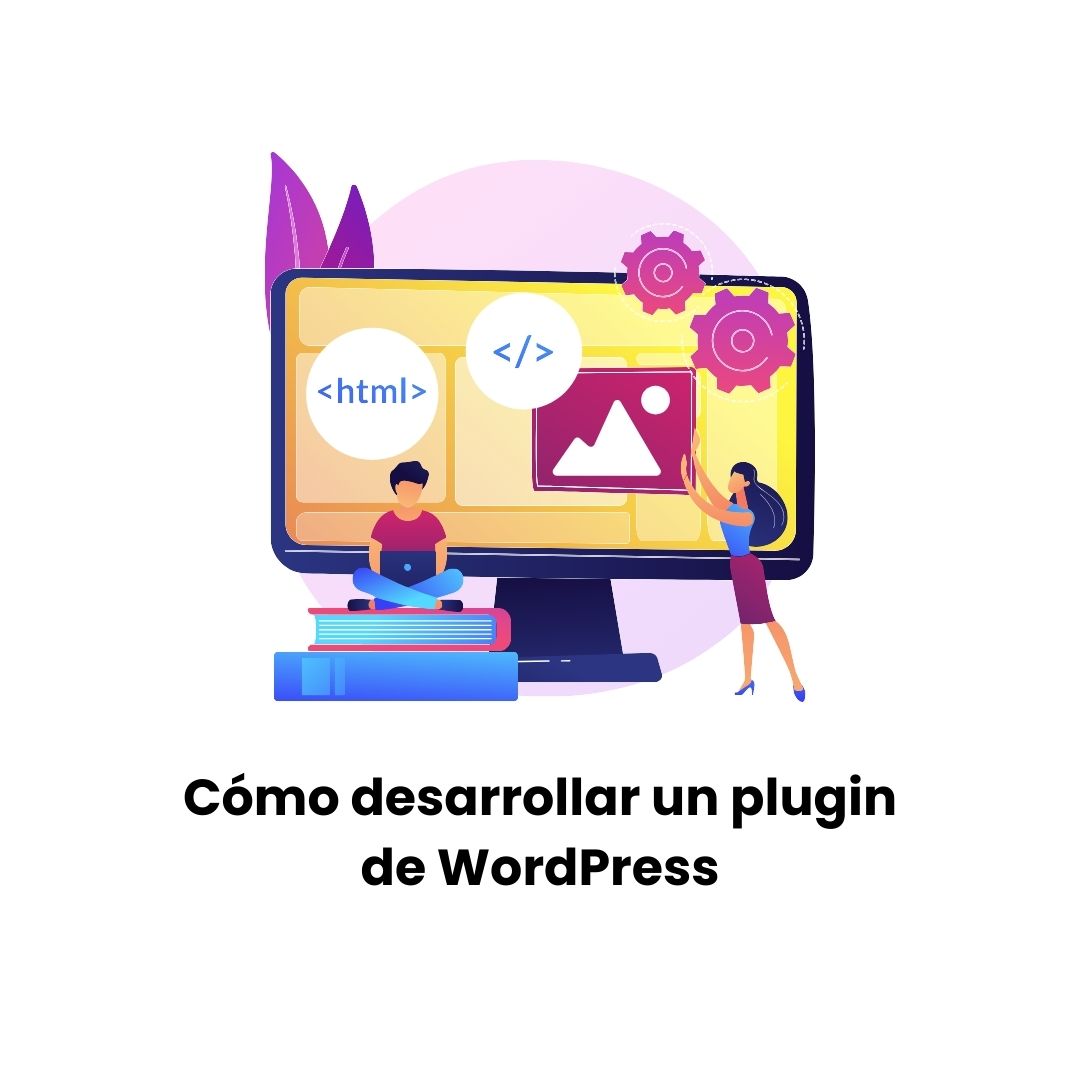
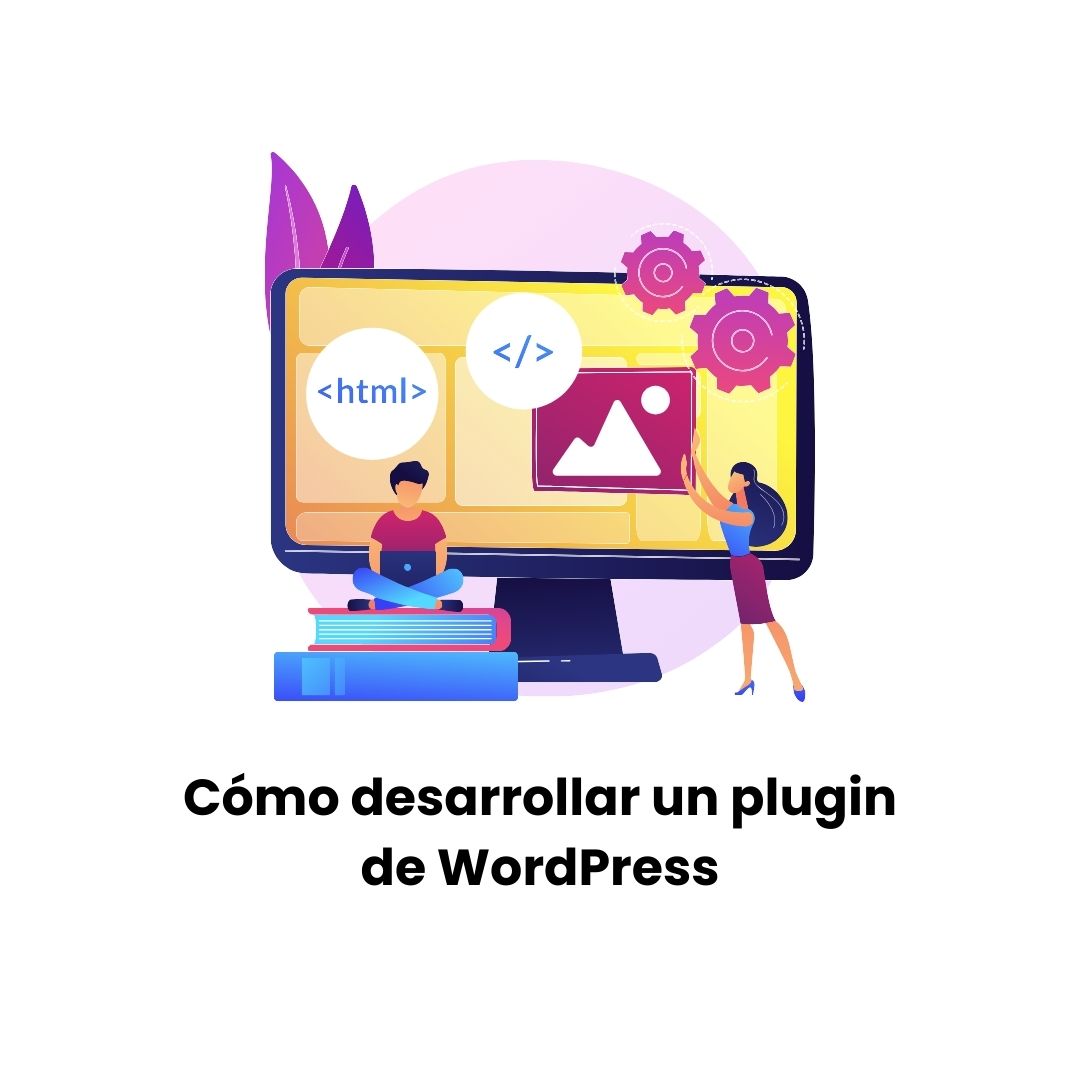
A WordPress plugin is a piece of software that integrates with the WordPress system to add new functionalities or enhance existing ones. Developing a plugin requires technical knowledge, but primarily, a clear understanding of what is to be achieved.
Contenidos
- 1 What is a WordPress plugin?
- 2 Benefits of using plugins
- 3 How plugins enhance your site
- 4 Before you start
- 5 What you need to know about PHP
- 5.1 Understanding WordPress structure
- 5.2 Plugin planning
- 5.3 Defining functionality
- 5.4 Market and competition analysis
- 5.5 Plugin development
- 5.6 Setting up the development environment
- 5.7 Creating the basic architecture of the plugin
- 5.8 Coding specific functionalities
- 5.9 Plugin testing
- 5.10 Unit testing
- 5.11 Integration testing
- 5.12 Launch and maintenance
- 5.13 Preparing for launch
- 5.14 Maintenance and update strategies
- 6 Programming a WordPress plugin step by step
- 6.1 Step 1:Set up your development environment
- 6.2 Step 2: Create a folder for your plugin
- 6.3 Step 3: Create the main file of the plugin
- 6.4 Step 4: Write the basic functionality of the plugin
- 6.5 Step 5: Activate your plugin
- 6.6 Step 6: Test your plugin
- 6.7 Step 7: Add more functionalities
- 6.8 Step 8: Prepare your plugin for distribution
- 6.9 Step 9: Upload your plugin to WordPress.org
- 6.10 Step 10: Maintenance and updates
What is a WordPress plugin?
WordPress plugins allow users to extend the capabilities of their websites simply and efficiently, without needing to alter the core code of the system.
Benefits of using plugins
Plugins offer significant advantages such as customization, scalability, and the ability to add complex functionalities without intervening in the base code.
How plugins enhance your site
With plugins, you can improve the user experience, optimize the site for search engines, or even add e-commerce functionalities simply.
Before you start
It is essential to have a basic knowledge of PHP and understand how WordPress works at the code level.
What you need to know about PHP
PHP is the programming language in which WordPress is written. Knowing its fundamentals is crucial for developing an effective plugin.
Understanding WordPress structure
Understanding the architecture of WordPress, including themes and plugins, will allow you to better integrate your code.
Plugin planning
Before coding, it is essential to define what problem your plugin will solve and how it will differ from existing ones.
Defining functionality
Clarify what your plugin will do and plan the specific functions you will need to develop.
Market and competition analysis
Research other similar plugins and determine what you can offer that is unique or improves upon what is already available.
Plugin development
Development should follow a structured methodology to ensure that the plugin is stable and secure.
Setting up the development environment
Prepare your local or cloud development environment, ensuring it is a suitable testing space.
Creating the basic architecture of the plugin
Start by establishing a solid file structure and follow WordPress coding best practices.
Coding specific functionalities
Develop the functions you defined in the planning phase, ensuring adherence to WordPress standards.
Plugin testing
Testing is essential to ensure that the plugin functions correctly in various environments and versions of WordPress.
Unit testing
Implement unit tests for each component of your plugin.
Integration testing
Perform integration tests to ensure that your plugin works well with other plugins and WordPress themes.
Launch and maintenance
Once the plugin is developed and tested, it is time to launch it and plan its maintenance.
Preparing for launch
Prepare everything necessary for a successful launch, including documentation and technical support.
Maintenance and update strategies
Plan how you will handle updates and long-term support to ensure that your plugin remains relevant and secure.
Programming a WordPress plugin step by step
Programming a WordPress plugin may seem like a daunting task, but by following a step-by-step guide, you can create a functional plugin that enhances the capabilities of your website or that of your clients. Below, I present the essential steps to develop a plugin from scratch:
Step 1:Set up your development environment
Before starting to write code, it is essential that you prepare a local development environment. This allows you to develop and test your plugin without affecting a live website. You can use tools like XAMPP, MAMP, or LocalWP to set up a local WordPress environment.
Step 2: Create a folder for your plugin
In your local WordPress installation, navigate to the wp-content/plugins folder and create a new folder for your plugin. The folder name should be descriptive and preferably without spaces, for example, my-first-plugin.
Step 3: Create the main file of the plugin
Inside the folder of your plugin, create a PHP file that will be the main file of your plugin. By convention, this file usually has the same name as the folder of the plugin, for example, my-first-plugin.php. Open this file in your code editor and add the following basic code:
phpCopy code<?php
/*
Plugin Name: My First Plugin
Plugin URI: http://yoursite.com
Description: This is my first WordPress plugin.
Version: 1.0
Author: Your Name
Author URI: http://yoursite.com
License: GPL2
*/
This header is essential, as it tells WordPress the key details about your plugin.
Step 4: Write the basic functionality of the plugin
Next, you can start writing the functions that will define the logic of your plugin. Suppose you want your plugin to simply display a message in all blog posts. You could add this code to the plugin file:
phpCopy codefunction show_message() {
return "<p>Message from My First Plugin</p>";
}
add_filter('the_content', 'show_message');
This code adds a filter that modifies the content of the posts, inserting a message at the end of each one.
Step 5: Activate your plugin
To see your plugin in action, you must activate it. Go to the WordPress admin area, navigate to the “Plugins” section, and activate “My First Plugin”.
Step 6: Test your plugin
Now that your plugin is active, navigate to any post on your blog to see the message that your plugin adds to the content. Conduct the necessary tests to ensure that everything works as expected.
Step 7: Add more functionalities
Now that you have the basic structure and your plugin is working, you can start to expand it. Depending on what you want to achieve, you can add new functions, create widgets, add shortcodes, or even integrate your plugin with external APIs.
Step 8: Prepare your plugin for distribution
If you plan to distribute your plugin to other WordPress users, make sure to follow the WordPress.org guidelines for plugins. This includes preparing good documentation, cleaning up the code, and ensuring that it complies with the security and coding standards of WordPress.
Step 9: Upload your plugin to WordPress.org
If you want your plugin to be available in the official plugin directory of WordPress, you can submit it for review. You will need to create an account on WordPress.org and follow the detailed submission process on their website.
Step 10: Maintenance and updates
Finally, after launching your plugin, it is important to keep it updated and respond to any issues that users may report. This includes updating your plugin to ensure that it is compatible with the latest versions of WordPress and fixing any bugs that may arise.
Knowing how to develop a WordPress plugin is an excellent way to improve your programming skills and contribute to the WordPress community. With practice and patience, you can create plugins that add significant functionalities to WordPress websites.
Example of a plugin created for WordPress I will provide you with a simple example of how you might create a plugin for WordPress that adds a specific functionality: a plugin that automatically adds a signature or personalized message at the end of each blog post. This type of plugin is useful for bloggers who want to maintain consistency in their posts or add a call to action at the end of each article.
Step 1: Create the plugin file First, you must create a PHP file for your plugin. Name this file, for example, signature-in-posts.php. This file should be located in the wp-content/plugins/signature-in-posts folder of your WordPress installation.
Step 2: Add the plugin header Every WordPress plugin needs a header that defines its basic metadata. Open the signature-in-posts.php file and add the following header:
phpCopy code<?php
/*
Plugin Name: Signature in Posts
Description: Adds a personalized signature at the end of each post.
Version: 1.0
Author: Your Name
*/
Step 3: Define the functionality of the plugin Next, you need to write the logic of the plugin. In this case, we want it to add text at the end of each blog post content. This can be done using a filter in WordPress. Here is an example of how to implement it:
phpCopy codefunction add_signature_to_posts($content) {
if (is_single() && is_main_query()) {
return $content . "<div class='signature-posts'>Thank you for reading my blog. Follow me on social media!</div>";
}
return $content;
}
add_filter('the_content', 'add_signature_to_posts');
In this code, add_signature_to_posts is a function that receives the content of the post as an argument, checks if the page is an individual post and if it is the main query (to avoid affecting other loops or widgets), and then adds the HTML of the signature at the end of the content.
Step 4: Activate the plugin Upload the signature-in-posts folder to your server in the wp-content/plugins folder. Go to the WordPress admin area and activate the plugin “Signature in Posts”. Step 5: Verify that it works Create or edit a post to see how the signature appears at the end of it. You can customize the signature message and CSS style according to your needs.
Additional considerations It’s a good idea to add some CSS to style the signature. You can do this by adding styles directly in the plugin file, using wp_enqueue_style, or adding them in the style sheet of your theme.
This basic example shows you how to start developing your own WordPress plugins, adding specific functionalities and learning to manipulate the contents of your website programmatically.